A user field in a list allows people to choose a user for each list item.
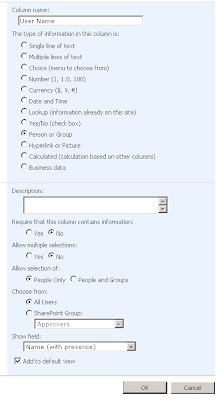
When looking at the value that is stored, we can see it is a lookup field - and is stored using the format ID#useraccount. For example:
listitem["User Name"] will have "1#development\ishai"
Click on the image below to see it in action in visual studio:
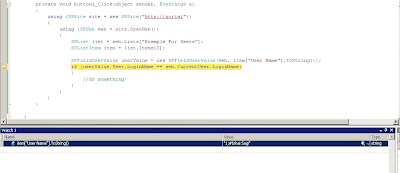
Where does this number come from? well, everytime you create a site in sharepoint, sharepoint creates a list for users. Each user gets an ID in the list, and when you add a user column to a list in that site, it is infact a special case of a lookup column into the users list. So in each site the same user will have a different ID.
So how do we use this field in code?
Get a user from a list item:
To get a user we use the SPFieldUserValue class, which accepts a SPWeb and a string value as parameters. The string value is the value from the list that contains the ID and the account name. Once we have that, we can use the SPFieldUserValue to get information about the user.
Example:
using (SPSite site = new SPSite("http://portal"))
{
using (SPWeb web = site.OpenWeb())
{
SPList list = web.Lists["Example For Users"];
SPListItem item = list.Items[0];
SPFieldUserValue userValue = new SPFieldUserValue(web, item["User Name"].ToString());
if (userValue.User.LoginName == web.CurrentUser.LoginName)
{
//do something!
}
}
}
Adding a value to a user field
This is the same, but in reverse. We use the same class (SPFieldUserValue ) and give it the values we want for the user.
using (SPSite site = new SPSite("http://portal"))
{
using (SPWeb web = site.OpenWeb())
{
SPList list = web.Lists["Example For Users"];
SPListItem item = list.Items[0];
SPFieldUserValue userValue = new SPFieldUserValue(web, web.CurrentUser.ID, web.CurrentUser.LoginName);
item["User Name"] = userValue;
item.Update();
}
}
The image below shows the list after I updated the list item:

'Solution Platform' 카테고리의 다른 글
Deleting a Page Layout or Master Page that is no longer referenced (0) | 2009.04.10 |
---|---|
Configuring Forms Authentication in SharePoint 2007 (0) | 2009.03.31 |
Complete reference of all STSADM operations (with parameters) in MOSS 2007 (0) | 2009.03.31 |
Silverlight & MOSS Web.Config Settings (0) | 2009.03.30 |
Hosting a WCF service in Windows Sharepoint Services v3.0 (1) | 2009.03.30 |